Introduction to version 5
In this guide, we're going to build a very simple React App, which will make use of ReactGrid.
NPM Install
npm i @silevis/reactgrid@alpha
Unlike previous versions, there's no longer a need to import the CSS file separately.
Integrate ReactGrid into the App
To integrate ReactGrid into your application, configure the grid using rows, columns, and cells. However, if you provide only cells with specified rowIndex
and colIndex
, the rows and columns will be automatically generated. If you want to define specific row heights, column widths or row and column reordering, you should pass them explicitly to the ReactGrid component. Below is a detailed breakdown of how to set this up, including an example configuration file.
Understanding the Workflow
Let's create a simple example including the aggregation. The values of the cells in the Sum row and Total column will be automatically calculated from the values entered into the cells in the H1 and H1 columns.
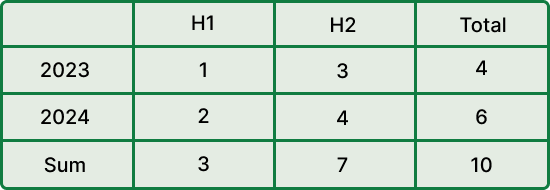
To achieve this, we will need an array of cells with defined indices, a template, and props. Once we have created all the necessary cells to build the example above, we can pass them to the ReactGrid component.
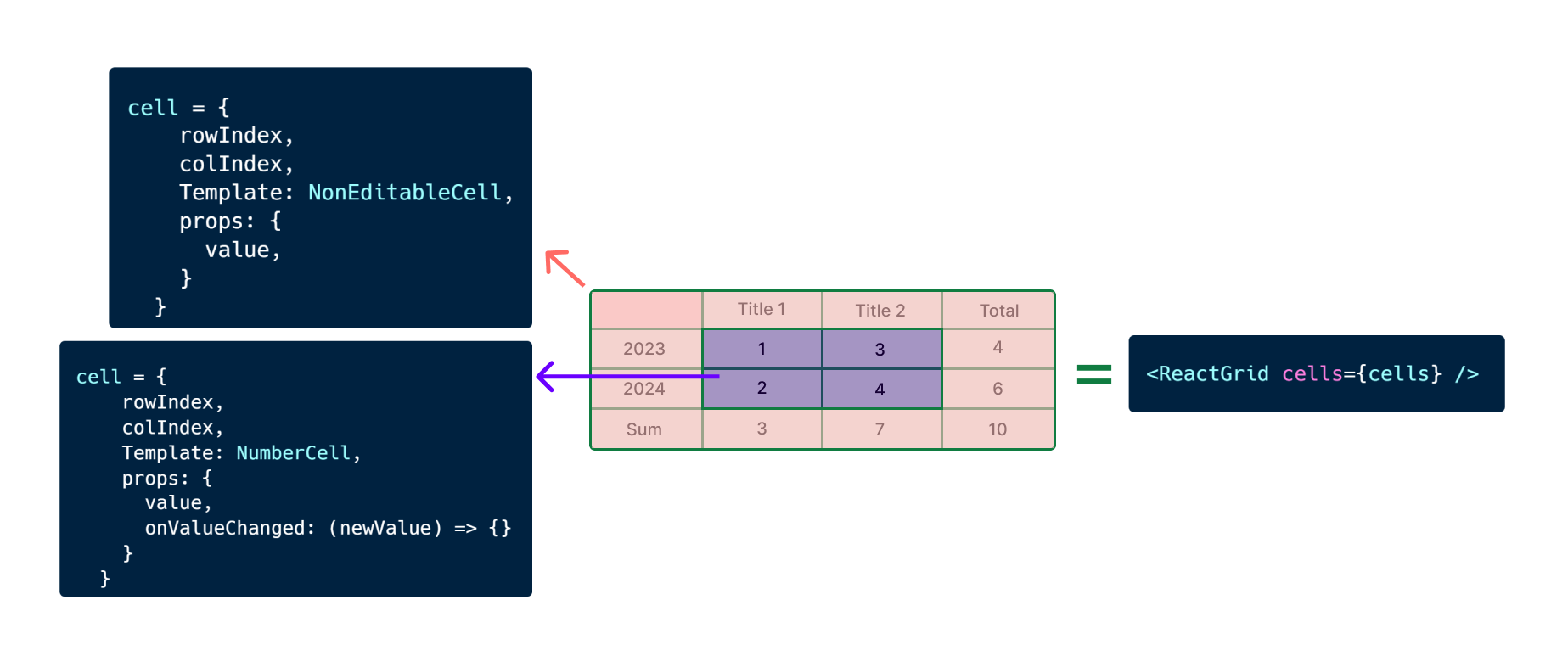
Live implementation
Code
const styledRanges = [ { range: { start: { rowIndex: 0, columnIndex: 1 }, end: { rowIndex: 1, columnIndex: 4 } }, styles: { background: "#55bc71", color: "white" }, }, { range: { start: { rowIndex: 1, columnIndex: 0 }, end: { rowIndex: 4, columnIndex: 1 } }, styles: { background: "#55bc71", color: "white" }, }, { range: { start: { rowIndex: 0, columnIndex: 0 }, end: { rowIndex: 1, columnIndex: 1 } }, styles: { background: "#318146", color: "white" }, }, { range: { start: { rowIndex: 3, columnIndex: 1 }, end: { rowIndex: 4, columnIndex: 4 } }, styles: { background: "#f8f8f8" }, }, { range: { start: { rowIndex: 1, columnIndex: 3 }, end: { rowIndex: 3, columnIndex: 4 } }, styles: { background: "#f8f8f8" }, }, ]; const ReactGridExample = () => { const [yearsData, setYearsData] = useState([ { label: "2023", values: [1, 3] }, { label: "2024", values: [2, 4] }, ]); // [{ label: "2023", values: [1, 3, 4] }, { label: "2024", values: [2, 4, 6] }] const yearsDataWithTotals = yearsData.map((year) => ({ ...year, values: [...year.values, year.values.reduce((sum, value) => sum + value, 0)], })); // { label: "Sum", values: [3, 7, 10] } const summaryRow = { label: "Sum", values: yearsDataWithTotals.reduce( (sum, year) => sum.map((currentSum, index) => currentSum + year.values[index]), [0, 0, 0] ), }; const cells: Cell[] = [ // Header cells { rowIndex: 0, colIndex: 0, Template: NonEditableCell, props: { value: "" } }, { rowIndex: 0, colIndex: 1, Template: NonEditableCell, props: { value: "H1" } }, { rowIndex: 0, colIndex: 2, Template: NonEditableCell, props: { value: "H2" } }, { rowIndex: 0, colIndex: 3, Template: NonEditableCell, props: { value: "Total" } }, // Year data cells ...yearsDataWithTotals .map((year, rowIndex) => [ { rowIndex: rowIndex + 1, colIndex: 0, Template: NonEditableCell, props: { value: year.label } }, ...year.values.map((value, colIndex) => { // Last column is not editable const isEditable = colIndex + 1 !== year.values.length; return { rowIndex: rowIndex + 1, colIndex: colIndex + 1, Template: !isEditable ? NonEditableCell : NumberCell, props: { value, ...(isEditable && { onValueChanged: (newValue) => { setYearsData((prev) => { const updatedYears = [...prev]; updatedYears[rowIndex].values[colIndex] = newValue; return updatedYears; }); }, }), }, }; }), ]) .flat(), // Summary row { rowIndex: yearsDataWithTotals.length + 1, colIndex: 0, Template: NonEditableCell, props: { value: summaryRow.label }, }, ...summaryRow.values.map((value, colIndex) => ({ rowIndex: yearsDataWithTotals.length + 1, colIndex: colIndex + 1, Template: NonEditableCell, props: { value }, })), ]; return <ReactGrid cells={cells} styledRanges={styledRanges} />; }; render(<ReactGridExample />, document.getElementById("root"));
Preview
Summary
You should now have a basic understanding of what ReactGrid is and how it can be used.